In this Open weather map API NodeJS tutorial, we are going to see how we can create a weather api with node js that will give us weather forecasts of any city/country. Seems Amazing right? So, without any further delay, let’s get started. We are going to use Open Weather Map API for fetching forecasting details of about 200,000 plus cities. You can read more about Open Weather Map API at Open Weather Map API.
Setting up developer account at Open Weather Map API.
For fetching details about the weather using the open weather map API, we will have to create a free account here. It’s quite simple, just follow the following steps.
- Go to this site Open Weather Map API and create an account. In case you already have an account, simply login.
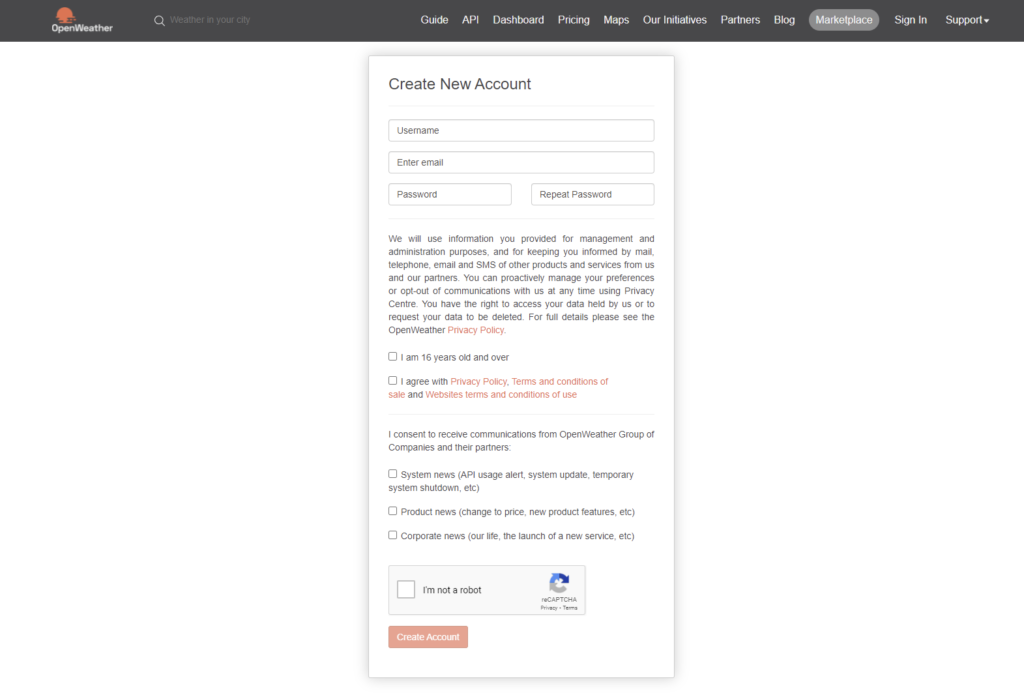
- After creating an account, you need to verify your email. And then simply login. You will see following dashboard under API section.
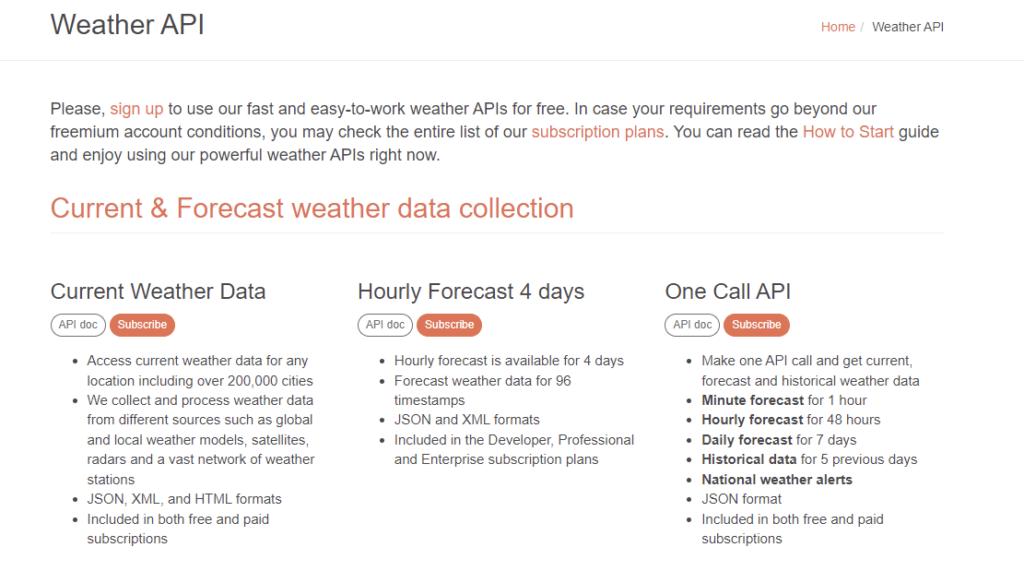
- We need to head over My API Keys section in nav bar under User Avatar.
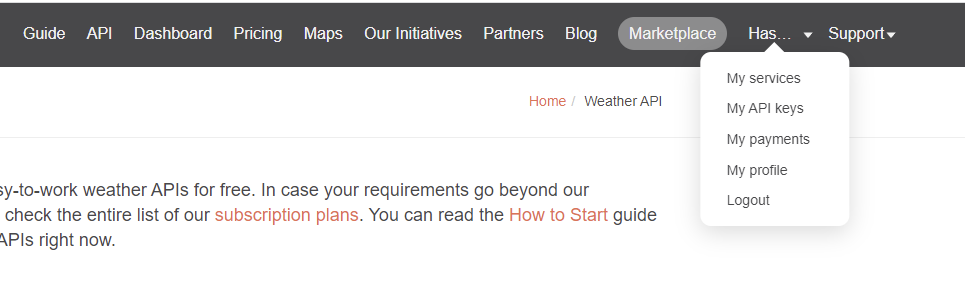
- Then give API key a name and click on create Key.
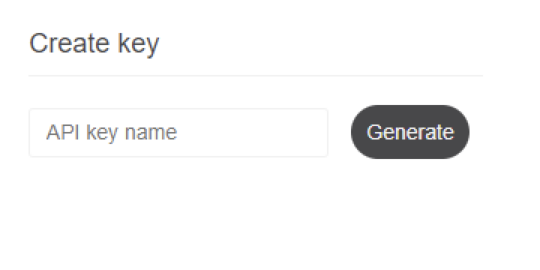
- Copy API key and let’s get started by creating node project.
- Create an empty directory, name it as u want. I am going to name it as weather-app
- Open it with vs code. You can download vs code from here. https://code.visualstudio.com/
- Go to terminal by pressing ctrl + shift + ~ and type npm init -y and hit enter.
- Head to package.json file and do the following changes.
{
"name": "weather-app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"type": "module",
"scripts": {
"start": "nodemon server.js"
},
"keywords": [],
"author": "",
"license": "ISC"
}
- Go to terminal and type npm install express cors dotenv node-fetch and hit enter
- Create an empty file server.js and put the following code inside it.
import express from "express";
import cors from "cors";
import dotenv from "dotenv";
import fetch from "node-fetch";
const app = express();
const port = process.env.PORT || 5000;
//registering middlewares
dotenv.config();
app.use(express.json());
app.use(cors());
//registering routes
app.get("/", (req, res) => {
res.status(200).send("Weather API is running");
});
//creating server
app.listen(port, () => {
console.log(`server is up on ${port}`);
});
Type npm start inside the terminal and congratulations, your server is running now.
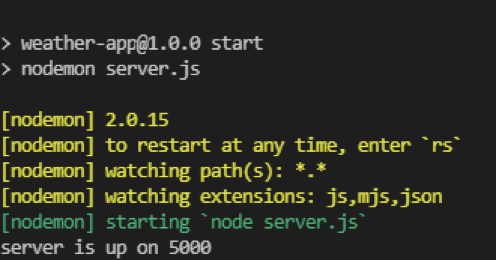
Now, remember that API Key you have copied from an open weather map. Create a .env file in your root directory and paste the following content.

Now all set, we are now going to use the following URL http://api.openweathermap.org/data/2.5/weather for making weather requests. Two query parameters are also required in the URL. Query parameters are key-value pairs that let us pass information to a URL. In this case, we’ll need to supply our API Key as well as the city we’re looking for. Let’s add a route for fetching weather forecasts for a particular city.
//fetching weather forecast for a particular city
app.get("/city", async (req, res) => {
if (!req.query.city) {
res.status(404).json("City is missing");
} else {
let city = req.query.city;
const response = await fetch(
`http://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${process.env.API_KEY}`
);
const data = await response.json();
res.status(200).json(data);
}
});
Our server.js looks as follows now.
import express from "express";
import cors from "cors";
import dotenv from "dotenv";
import fetch from "node-fetch";
const app = express();
const port = process.env.PORT || 5000;
//registering middlewares
dotenv.config();
app.use(express.json());
app.use(cors());
//registering routes
app.get("/", (req, res) => {
res.status(200).send("Weather API is running");
});
//fetching weather forecast for a particular city
app.get("/weather", async (req, res) => {
if (!req.query.city) {
res.status(404).json("City is missing");
} else {
let city = req.query.city;
const response = await fetch(
`http://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${process.env.API_KEY}`
);
const data = await response.json();
res.status(200).json(data);
}
});
//creating server
app.listen(port, () => {
console.log(`server is up on ${port}`);
});
Output
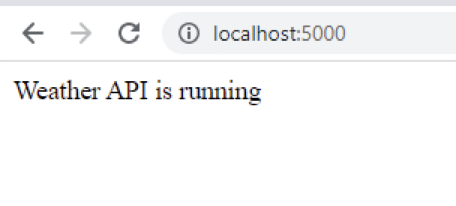

The weather data.
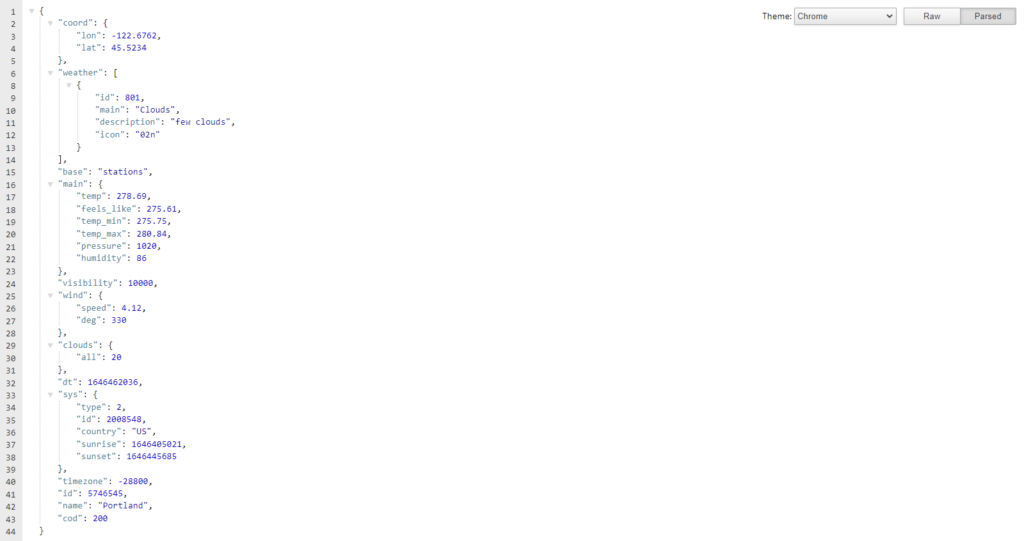
Conclusion
We have created our own weather API with node js. I hope you enjoyed this simple yet powerful node js weather API. You can read more about weather API here Open Weather Map API and stay connected with nodejstutor for more tutorials.