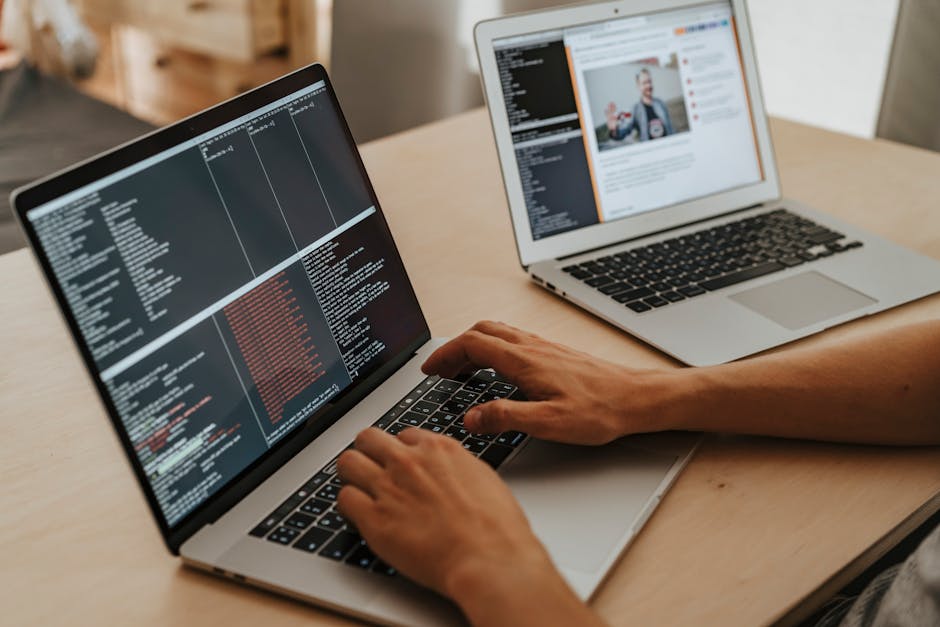
In the previous tutorial, we covered the basic concepts and introduction to Node JS and environment setup. In this article, you will learn how to create a Node JS application (which outputs “Hello World“) and run it in the browser.
Before starting creating our first Node JS app, go through the components of the JS application.
Components of Node JS application
Required module- There are many important Node JS modules that need to be included in the project. We use the required directive to Import these modules.
Server – Server is also a major component of Node JS. It listens to the client requests and returns responses. If you have an idea about the apache server, you can use that idea for the time being to understand the Node JS server.
Read Request – The client can make request to the node server either through the browser, some API client like Postman, or console. The Node JS server is responsible to listen to these requests/
Return Response – The Node JS server process the request received from the client-side and returns the response to it.
How to create an application in Node JS
In this section, you will learn how to create the first application in Node JS. We will try to keep the application as simple as possible.
Step 1. Create a new file
In the very first step, you need to open any code editor. Create a new file in it. Save the file with the .js extension.
We recommend using Visual Studio Code. VS Code is free to download and is provided by Microsoft. It has great support for Node JS and provides many built-in extensions that enhance the Node JS coding experience.
Step 2. Import required Module
Require is a directive in Node JS. It allows us to consume the Node JS, Node JS community, and even local modules available yet in it. Using the required directive, we import the HTTP module and store it in the variable.
var http = require("http");
Step 3. Creating a new Node JS server
Port: There are many processes running on the server. When a request arrives at the server, the port number is used to identify the process to which this request is assigned.
We have created an HTTP instance in the second step. Use this instance to call the createServer() method. After creating the server, we bind it with port 8081 which usually listens to the HTTP requests.
Listen method is used to bind the server to the port number.
Look at the following piece of code and practice at your computer.
http.createServer(function (request, response) {
// Send the HTTP header
// HTTP Status: 200 : OK
// Content Type: text/plain
response.writeHead(200, {'Content-Type': 'text/plain'});
// Send the response body as "Hello World"
response.end('Hello World\n');
}).listen(8081);
// Console will print the message
console.log('Server running at http://127.0.0.1:8081/');
The above piece of code will listen to the incoming HTTP request and respond to them over port number 8081.
Step 4. Request and Response Test in browser
We have created a server in the previous step. Save the file on your computer (for easy access, save it on the desktop with the name main.js). We print some messages in the console, that will help to verify the request and response. Let’s check the following code.
var http = require("http");
http.createServer(function (request, response) {
// Send the HTTP header
// HTTP Status: 200 : OK
// Content Type: text/plain
response.writeHead(200, {'Content-Type': 'text/plain'});
// Send the response body as "Hello World"
response.end('Hello World\n');
}).listen(8081);
// Console will print the message
console.log('Server running at http://127.0.0.1:8081/');
Start the node server by running the following command.
$ node main.js
After the server has started, it will print the following message in the terminal.
Server running at http://127.0.0.1:8081/
Step 5. Run the application in the browser
Now open any web browser like Google Chrome and open the URL returned by running the node server in the previous step. http://127.0.0.1:8081/
It will print the request message Hello World. This means that the server is listening to the requests and responding correctly.
Done! You have successfully created your first Node JS project. In this article, we learned about the components of the Node project, which require directive, port, server, request, and response. You must try these concepts on your computer before moving to the next article.