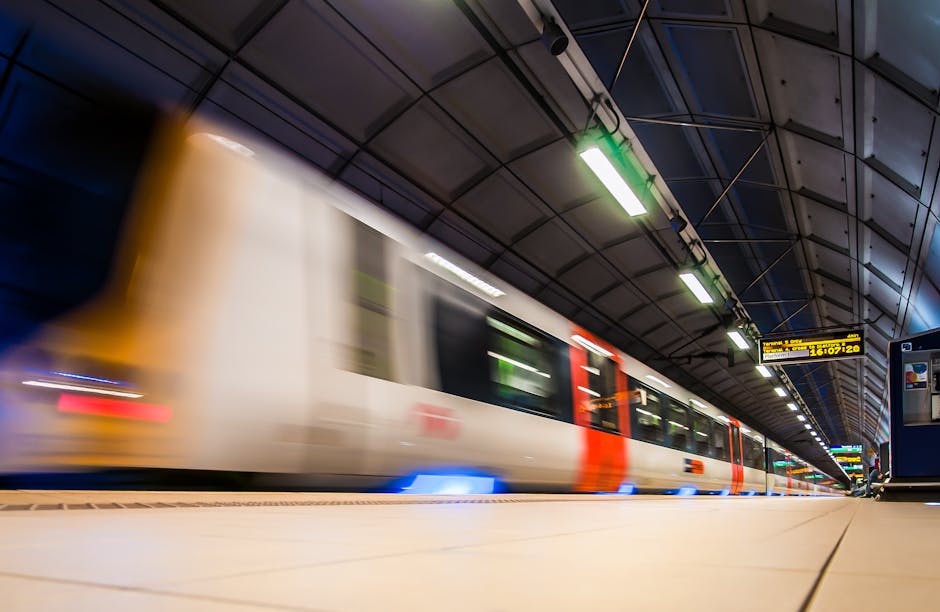
ExpressJS for NodeJS is a lightweight web framework. It offers a comprehensive set of functionality for online and mobile apps. ExpressJS is an excellent solution for both developers and companies due to its tiny and versatile core.
Installing ExpressJS
To install ExpressJS, use npm to install the framework (Node Package Manager). To install it, use the following command:
npm install express
Once you have installed ExpressJS, you can start building your web application. To get started, create a new file named app.js
and add the following code:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
Routing
Routing is an important element of ExpressJS. The process of determining how an application replies to a client request for a certain endpoint, which is a URI (or route) and a specific HTTP request type, is referred to as routing (GET, POST, and so on).
As illustrated in the code above, you can use the app.get() function to establish a new route. Other methods, such as app.post() and app.put(), can also be used to handle other HTTP methods.
Middleware
ExpressJS also has a robust middleware system, which runs functions before a request is processed. Middleware can help with functions like logging, authentication, and data validation.
Here’s an example of how middleware may be used in ExpressJS:
app.use((req, res, next) => {
console.log(`Request received at ${new Date().toLocaleString()}`);
next();
});
app.get('/', (req, res) => {
res.send('Hello, World!');
});
We’ve included a middleware function in this example that logs the date and time of each incoming request. The next() function is used to transfer control to the following middleware function or request handler.
Template Engine
ExpressJS includes a number of template engines for dynamically generating HTML, CSS, and JavaScript on the server. Pug, Mustache, and EJS are some prominent ExpressJS template engines.
Here’s how Pug may be used as a template engine in ExpressJS:
const express = require('express');
const app = express();
app.set('view engine', 'pug');
app.get('/', (req, res) => {
res.render('index', { title: 'My Express App' });
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
In this case, we’ve changed the view engine to Pug and added a new route that renders a Pug template. The template is saved in the index.pug file and should include the following code:
doctype html
html
head
title=title
body
h1 Hello, World!
Q&A
Q: What is ExpressJS used for?
A: ExpressJS is a fast and minimalist web framework for Node.js. It is used to build web and mobile applications.
Q: How do I install ExpressJS?
A: To install ExpressJS, you can use npm (Node Package Manager) by running the following command: npm install express
.
Q: What are some features of ExpressJS?
A: Some features of ExpressJS include routing, middleware, and support for template engines.
Exercises
- Create a new ExpressJS app that displays a “Welcome to my ExpressJS app” message.
- Add a new route to your ExpressJS app that displays a list of your favorite movies.
- Add a middleware function to your ExpressJS app that logs the current time for each incoming request.
Answers
1.
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Welcome to my ExpressJS app');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
2.
const express = require('express');
const app = express();
app.get('/movies', (req, res) => {
res.send(['The Matrix', 'Inception', 'The Shawshank Redemption']);
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
3.
const express = require('express');
const app = express();
app.use((req, res, next) => {
console.log(`Time: ${new Date().toLocaleString()}`);
next();
});
app.get('/', (req, res) => {
res.send('Welcome to my ExpressJS app');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});