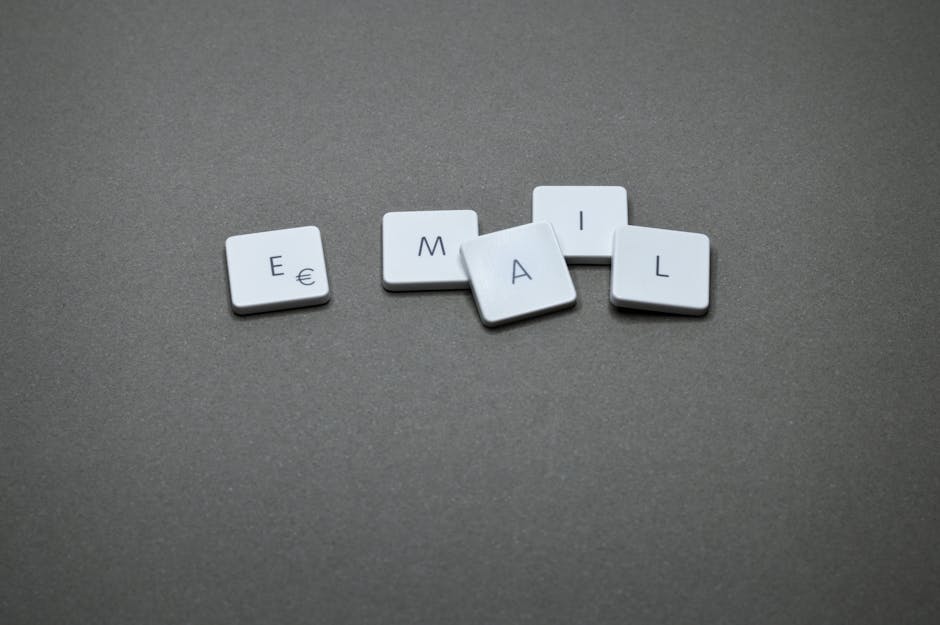
Email is one of the most popular forms of communication in the digital age. Many online applications require the ability to send and receive emails. NodeJS offers a variety of alternatives for delivering emails.
In this post, we’ll look at how to send email in NodeJS using several modules, including the built-in ‘nodemailer‘ module.
Sending emails
NodeJS has a number of modules for sending emails. The built-in ‘nodemailer’ module, on the other hand, is one of the most popular and commonly used modules. This module is simple to set up and use, and it includes a number of complex capabilities such as attachments, HTML content, and more.
Here is a basic example of sending an email using the ‘nodemailer’ module:
const nodemailer = require('nodemailer');
// create reusable transporter object using the default SMTP transport
const transporter = nodemailer.createTransport({
host: 'smtp.example.com',
port: 587,
secure: false, // true for 465, false for other ports
auth: {
user: 'username@example.com',
pass: 'userpassword'
}
});
// setup email data with unicode symbols
const mailOptions = {
from: '"Fred Foo 👻" <foo@example.com>', // sender address
to: 'bar@example.com, baz@example.com', // list of receivers
subject: 'Hello ✔', // Subject line
text: 'Hello world?', // plain text body
html: '<b>Hello world?</b>' // html body
};
// send mail with defined transport object
transporter.sendMail(mailOptions, (error, info) => {
if (error) {
return console.log(error);
}
console.log('Message sent: %s', info.messageId);
console.log('Preview URL: %s', nodemailer.getTestMessageUrl(info));
});
Explanation:
- The ‘nodemailer’ module is required in the first step.
- Then, using the default SMTP transport, we construct a transporter object and configure it with our email host and authentication information.
- Then we define email choices such the sender, recipients, topic, and message text.
- Finally, to send the email, we use the sendMail() method.
Q&A
Q: What is the ‘nodemailer’ module in NodeJS?
A: The ‘nodemailer’ module is a popular and widely used NodeJS module for sending emails. It provides advanced features, such as attachments, HTML content, and more.
Q: How do I send an email?
A: To send an email using NodeJS, you need to install and use a module, such as the built-in ‘nodemailer’ module. You can use the module to create a transporter object, configure it with your email host and authentication details, and then send the email using the sendMail() function.
Exercises
- Write a program to send a basic email from your NodeJS application.
- Write a program to receive emails from a specific email address using NodeJS.
- Write a program to send an HTML formatted email using NodeJS.
Answers
- Here is an example of sending a basic email using NodeJS:
const nodemailer = require('nodemailer');
let transporter = nodemailer.createTransport({
host: 'smtp.gmail.com',
port: 587,
secure: false,
auth: {
user: 'youremail@gmail.com',
pass: 'yourpassword'
}
});
let mailOptions = {
from: 'youremail@gmail.com',
to: 'recipientemail@gmail.com',
subject: 'Subject of the Email',
text: 'Hello, this is a test email sent from NodeJS.'
};
transporter.sendMail(mailOptions, function(error, info){
if (error) {
console.log(error);
} else {
console.log('Email sent: ' + info.response);
}
});
- Here is an example of receiving emails using NodeJS:
const imap = require('imap');
const simpleParser = require('mailparser').simpleParser;
let imapConfig = {
user: 'youremail@gmail.com',
password: 'yourpassword',
host: 'imap.gmail.com',
port: 993,
tls: true
};
let imapConnection = new imap(imapConfig);
imapConnection.once('ready', function() {
imapConnection.openBox('INBOX', true, function(error, box) {
if (error) throw error;
let searchCriteria = ['UNSEEN'];
let fetchOptions = {
bodies: ['HEADER', 'TEXT'],
markSeen: true
};
imapConnection.search(searchCriteria, fetchOptions, function(error, messages) {
if (error) throw error;
messages.forEach(function(message) {
simpleParser(message.parts[1].body, function(error, email) {
console.log(email.text);
});
});
});
});
});
imapConnection.connect();
- Here is an example of sending an HTML formatted email using NodeJS:
var nodemailer = require('nodemailer');
var transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'youremail@gmail.com',
pass: 'yourpassword'
}
});
var mailOptions = {
from: 'youremail@gmail.com',
to: 'recipient1@example.com, recipient2@example.com, recipient3@example.com',
subject: 'Sending Email using Node.js',
text: 'That was easy!'
};
transporter.sendMail(mailOptions, function(error, info){
if (error) {
console.log(error);
} else {
console.log('Email sent: ' + info.response);
}
});
In this answer above, we are sending the same email to three recipients recipient1@example.com
, recipient2@example.com
, and recipient3@example.com
. The nodemailer
library will handle the process of sending an email to multiple recipients.