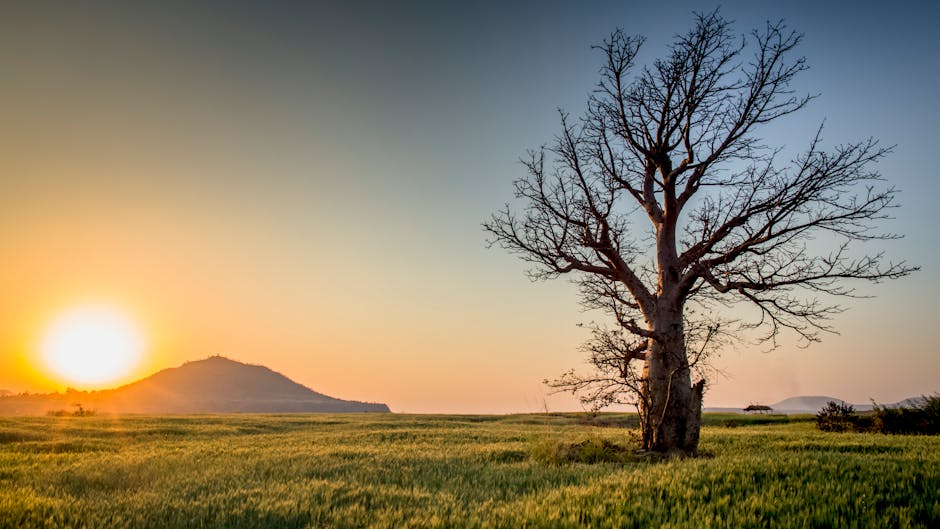
KoaJS is a contemporary framework for NodeJS that makes it easy to create online apps. It is based on Node.js and is intended to be tiny, expressive, and minimalistic. KoaJS is a middleware-based design that enables adding additional functionality to your online application simple. This framework is extensively used by developers who wish to create web applications that are quick, scalable, and efficient.
Middleware
KoaJS is a middleware-based framework that allows you to customize your web application’s functionality. In KoaJS, middleware functions get the context of a request and response. The sequence in which middleware functions are introduced to the application determines how they are run.
Examples of Middleware Functions:
- The code below shows how to use KoaJS to add logging capability to your web application.
const Koa = require('koa');
const app = new Koa();
app.use(async (ctx, next) => {
console.log(`Processing request for ${ctx.request.path}`);
await next();
});
Explanation: The middleware function logs the URL of the incoming request. The next() function is called to continue processing the request.
2. Body Parser
The code below shows how to add a body parser to your web application using KoaJS.
const Koa = require('koa');
const bodyParser = require('koa-bodyparser');
const app = new Koa();
app.use(bodyParser());
Explanation: The bodyParser() function is added as middleware to parse the request body and make it available as a property of the context object.
Q&A
Q:What is KoaJS?
A: KoaJS is a modern Node.js framework that offers a simple and elegant way to build web applications.
Q: What are middleware functions in KoaJS?
A: Middleware functions in KoaJS are functions that receive the context of a request and response. Middleware functions are executed in the order in which they are added to the application.
Q: How do I add custom functionality to my web application using KoaJS?
A: You can add custom functionality to your web application using KoaJS by adding middleware functions. Middleware functions receive the context of a request and response and can add custom functionality to your web application.
Exercises
- Create a KoaJS application that returns “Hello World!”
- Create a KoaJS application that returns the request URL.
Answers
1.
const Koa = require('koa');
const app = new Koa();
app.use(async (ctx, next) => {
ctx.body = "Hello World!";
await next();
});
app.listen(3000);
2.
const Koa = require('koa');
const app = new Koa();
app.use(async (ctx, next) => {
ctx.body = ctx.request.url;
await next();
});
app.listen(3000);